Good tools make application development quicker and easier to maintain than if you did everything by hand.
好的工具能让开发更加简单快捷。
The Angular CLI is a command line interface tool that can create a project, add files, and perform a variety of ongoing development tasks such as testing, bundling, and deployment.
Angular CLI是一个命令行界面工具,它可以创建项目、添加文件以及执行一大堆开发任务,比如测试、打包和发布。
The goal in this guide is to build and run a simple Angular application in TypeScript, using the Angular CLI while adhering to the Style Guide recommendations that benefit every Angular project.
在这一章CLI快速起步中,我们的目标是构建并运行一个超级简单的Angular应用。我们会使用Angular-CLI来让每个Angular应用从风格指南中获益。
By the end of the chapter, you'll have a basic understanding of development with the CLI and a foundation for both these documentation samples and for real world applications.
在本章的末尾,我们会通过CLI对开发过程有一个最基本的理解,并将其作为其它文档范例以及真实应用的基础。
You'll pursue these ends in the following high-level steps:
我们通过下列三大步来达到目的:
Set up the development environment.
设置开发环境。
Create a new project and skeleton application.
创建新项目以及应用的骨架。
Serve the application.
启动开发服务器。
Edit the application.
编辑该应用。
And you can also download the example.
你还可以下载这个例子。
Step 1. Set up the Development Environment
步骤1. 设置开发环境
You need to set up your development environment before you can do anything.
在开始工作之前,我们必须设置好开发环境。
Install Node.js® and npm if they are not already on your machine.
如果你的机器上还没有Node.js®和npm,请先安装它们。
Verify that you are running at least node 6.9.x
and npm 3.x.x
by running node -v
and npm -v
in a terminal/console window.
Older versions produce errors, but newer versions are fine.
请先在终端/控制台窗口中运行命令 node -v
和 npm -v
,
来验证一下你正在运行 node 6.9.x
和 npm 3.x.x
以上的版本。
更老的版本可能会出现错误,更新的版本则没问题。
Then install the Angular CLI globally.
然后全局安装 Angular CLI 。
Step 2. Create a new project
步骤2. 创建新项目
Open a terminal window.
打开终端窗口。
Generate a new project and skeleton application by running the following commands:
运行下列命令来生成一个新项目以及应用的骨架代码:
Patience please. It takes time to set up a new project, most of it spent installing npm packages.
请耐心等待。 创建新项目需要花费很多时间,大多数时候都是在安装那些npm包。
Step 3: Serve the application
步骤3. 启动开发服务器
Go to the project directory and launch the server.
进入项目目录,并启动服务器。
The ng serve
command launches the server, watches your files,
and rebuilds the app as you make changes to those files.
ng serve
命令会启动开发服务器,监听文件变化,并在修改这些文件时重新构建此应用。
Using the --open
(or just -o
) option will automatically open your browser
on http://localhost:4200/
.
使用--open
(或-o
)参数可以自动打开浏览器并访问http://localhost:4200/
。
Your app greets you with a message:
本应用会用一条消息来跟你打招呼:
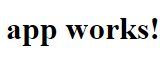
Step 4: Edit your first Angular component
步骤4. 编辑我们的第一个Angular组件
The CLI created the first Angular component for you.
This is the root component and it is named app-root
.
You can find it in ./src/app/app.component.ts
.
这个CLI为我们创建了第一个Angular组件。
它就是名叫app-root
的根组件。
你可以在./src/app/app.component.ts
目录下找到它。
Open the component file and change the title
property from app works! to My First Angular App:
打开这个组件文件,并且把title
属性从 app works! 改为 My First Angular App :
src/app/app.component.ts
The browser reloads automatically with the revised title. That's nice, but it could look better.
浏览器会自动刷新,而我们会看到修改之后的标题。不错,不过它还可以更好看一点。
Open src/app/app.component.css
and give the component some style.
打开 src/app/app.component.css
并给这个组件设置一些样式
src/app/app.component.css

Looking good!
漂亮!
What's next?
接下来呢?
That's about all you'd expect to do in a "Hello, World" app.
如你所愿,我们完成了这个“Hello, World”应用。
You're ready to take the Tour of Heroes Tutorial and build a small application that demonstrates the great things you can build with Angular.
现在,你可以开始英雄指南教程,通过构建一个小型应用来学习如何用Angular构建各种大型应用了。
Or you can stick around a bit longer to learn about the files in your brand new project.
或者,你也可以稍等一会儿,学学在这个新项目中的文件都是干什么用的。
Project file review
项目文件概览
An Angular CLI project is the foundation for both quick experiments and enterprise solutions.
Angular CLI项目是做快速试验和开发企业解决方案的基础。
The first file you should check out is README.md
.
It has some basic information on how to use CLI commands.
Whenever you want to know more about how Angular CLI works make sure to visit
the Angular CLI repository and
Wiki.
你首先要看的文件是README.md
。
它提供了一些如何使用CLI命令的基础信息。
如果你想了解 Angular CLI 的工作原理,请访问 Angular CLI 的仓库及其
Wiki。
Some of the generated files might be unfamiliar to you.
有些生成的文件你可能觉得陌生。接下来我们就讲讲它们。
The src
folder
src
文件夹
Your app lives in the src
folder.
All Angular components, templates, styles, images, and anything else your app needs go here.
Any files outside of this folder are meant to support building your app.
你的应用代码位于src
文件夹中。
所有的Angular组件、模板、样式、图片以及你的应用所需的任何东西都在那里。
这个文件夹之外的文件都是为构建应用提供支持用的。
File 文件 | Purpose 用途 |
---|---|
app/app.component.{ts,html,css,spec.ts} | Defines the 使用HTML模板、CSS样式和单元测试定义 |
app/app.module.ts | Defines 定义 |
assets/* | A folder where you can put images and anything else to be copied wholesale when you build your application. 这个文件夹下你可以放图片等任何东西,在构建应用时,它们全都会拷贝到发布包中。 |
environments/* | This folder contains one file for each of your destination environments, each exporting simple configuration variables to use in your application. The files are replaced on-the-fly when you build your app. You might use a different API endpoint for development than you do for production or maybe different analytics tokens. You might even use some mock services. Either way, the CLI has you covered. 这个文件夹中包括为各个目标环境准备的文件,它们导出了一些应用中要用到的配置变量。 这些文件会在构建应用时被替换。 比如你可能在产品环境中使用不同的API端点地址,或使用不同的统计Token参数。 甚至使用一些模拟服务。 所有这些,CLI都替你考虑到了。 |
favicon.ico | Every site wants to look good on the bookmark bar. Get started with your very own Angular icon. 每个网站都希望自己在书签栏中能好看一点。 请把它换成你自己的图标。 |
index.html | The main HTML page that is served when someone visits your site.
Most of the time you'll never need to edit it.
The CLI automatically adds all 这是别人访问你的网站是看到的主页面的HTML文件。
大多数情况下你都不用编辑它。
在构建应用时,CLI会自动把所有 |
main.ts | The main entry point for your app.
Compiles the application with the JIT compiler
and bootstraps the application's root module ( 这是应用的主要入口点。
使用JIT compiler编译器编译本应用,并启动应用的根模块 |
polyfills.ts | Different browsers have different levels of support of the web standards.
Polyfills help normalize those differences.
You should be pretty safe with 不同的浏览器对Web标准的支持程度也不同。
填充库(polyfill)能帮我们把这些不同点进行标准化。
你只要使用 |
styles.css | Your global styles go here. Most of the time you'll want to have local styles in your components for easier maintenance, but styles that affect all of your app need to be in a central place. 这里是你的全局样式。 大多数情况下,你会希望在组件中使用局部样式,以利于维护,不过那些会影响你整个应用的样式你还是需要集中存放在这里。 |
test.ts | This is the main entry point for your unit tests. It has some custom configuration that might be unfamiliar, but it's not something you'll need to edit. 这是单元测试的主要入口点。 它有一些你不熟悉的自定义配置,不过你并不需要编辑这里的任何东西。 |
tsconfig.{app|spec}.json | TypeScript compiler configuration for the Angular app ( TypeScript编译器的配置文件。 |
The root folder
根目录
The src/
folder is just one of the items inside the project's root folder.
Other files help you build, test, maintain, document, and deploy the app.
These files go in the root folder next to src/
.
src/
文件夹是项目的根文件夹之一。
其它文件是用来帮助你构建、测试、维护、文档化和发布应用的。它们放在根目录下,和src/
平级。
File 文件 | Purpose 用途 |
---|---|
e2e/ | Inside 在 |
node_modules/ |
|
.angular-cli.json | Configuration for Angular CLI. In this file you can set several defaults and also configure what files are included when your project is build. Check out the official documentation if you want to know more. Angular CLI的配置文件。 在这个文件中,我们可以设置一系列默认值,还可以配置项目编译时要包含的那些文件。 要了解更多,请参阅它的官方文档。 |
.editorconfig | Simple configuration for your editor to make sure everyone that uses your project
has the same basic configuration.
Most editors support an 给你的编辑器看的一个简单配置文件,它用来确保参与你项目的每个人都具有基本的编辑器配置。
大多数的编辑器都支持 |
.gitignore | Git configuration to make sure autogenerated files are not commited to source control. 一个Git的配置文件,用来确保某些自动生成的文件不会被提交到源码控制系统中。 |
karma.conf.js | Unit test configuration for the Karma test runner,
used when running 给Karma的单元测试配置,当运行 |
package.json |
|
protractor.conf.js | End-to-end test configuration for Protractor,
used when running 给Protractor使用的端到端测试配置文件,当运行 |
README.md | Basic documentation for your project, pre-filled with CLI command information. Make sure to enhance it with project documentation so that anyone checking out the repo can build your app! 项目的基础文档,预先写入了CLI命令的信息。 别忘了用项目文档改进它,以便每个查看此仓库的人都能据此构建出你的应用。 |
tsconfig.json | TypeScript compiler configuration for your IDE to pick up and give you helpful tooling. TypeScript编译器的配置,你的IDE会借助它来给你提供更好的帮助。 |
tslint.json | Linting configuration for TSLint together with
Codelyzer, used when running 给TSLint和Codelyzer用的配置信息,当运行 |
Next Step
下一步
If you're new to Angular, continue on the learning path. You can skip the "Setup" step since you're already using the Angular CLI setup.
如果你刚刚开始使用Angular,我们建议你遵循这个学习路径。 你可以跳过“环境设置”一章,因为你已经在使用 Angular-CLI 设置好环境了。